用vue3 和ts, 写这个图片的界面,完全不依赖任何UI框架,完全自定义组件实现的站点导航系统,包含左侧分类导航、搜索功能和右侧iframe展示。 左边是一个多分类tab的站点导航,右边是一个iframe框展示左边的内容,并且把代码打包下载给我,不要使用框架,用自定义组件的方式写,左边导航有个搜索框,可用搜索json数据,数据是json文件从服务器一次性加载,分类和站点点击增加着色,默认界面,鼠标移动到分类上面显示一个弹出层显示站点列表,分类右侧中间位置有一个展开按钮,点击按钮,站点列表变成固定在分类右侧,形成左中右布局,要求代码简洁高效,防止鼠标移动出现闪烁。
用vue3 和ts, 写这个图片的界面,完全不依赖任何UI框架,完全自定义组件实现的站点导航系统,包含左侧分类导航、搜索功能和右侧iframe展示。 左边是一个多分类tab的站点导航,右边是一个iframe框展示左边的内容,并且把代码打包下载给我,不要使用框架,用自定义组件的方式写,左边导航有个搜索框,可用搜索json数据,数据是json文件从服务器一次性加载,分类和站点点击增加着色,默认界面,鼠标移动到分类上面显示一个弹出层显示站点列表,分类右侧中间位置有一个展开按钮,点击按钮,站点列表变成固定在分类右侧,形成左中右布局,要求代码简洁高效,防止鼠标移动出现闪烁。
用vue3 和ts, 写这个图片的界面,完全不依赖任何UI框架,完全自定义组件实现的站点导航系统,包含左侧分类导航、搜索功能和右侧iframe展示。 左边是一个多分类tab的站点导航,右边是一个iframe框展示左边的内容,并且把代码打包下载给我,不要使用框架,用自定义组件的方式写,左边导航有个搜索框,可用搜索json数据,数据是json文件从服务器一次性加载,分类和站点点击增加着色,默认界面,鼠标移动到分类上面显示一个弹出层显示站点列表,分类右侧中间位置有一个展开按钮,点击按钮,站点列表变成固定在分类右侧,形成左中右布局,要求代码简洁高效,防止鼠标移动出现闪烁。
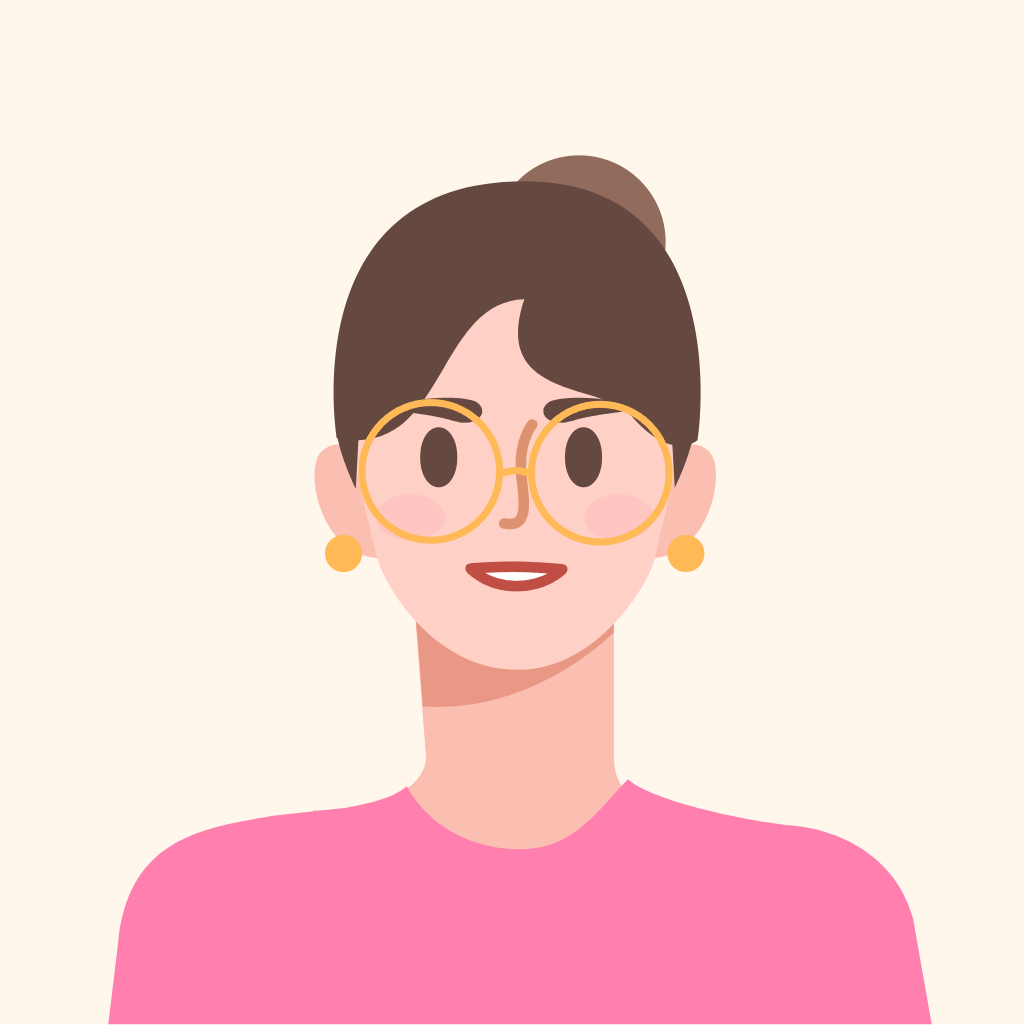
用户464642096
05月17日 21:18
|
浏览 39
好的,下面是一个基于 Vue 3 和 TypeScript 的自定义组件实现的简单站点导航系统示例。这个示例包括左侧分类导航、搜索功能和右侧 iframe 展示。我们将使用 Vue 3 的 Composition API 来实现自定义组件。
目录结构
复制代码
src/
├── components/
│ ├── LeftNav.vue
│ ├── SearchBar.vue
│ └── ExpandButton.vue
├── App.vue
├── main.ts
├── data.json
数据文件 (data.json)
复制代码
{
"categories": [
{
"name": "Category 1",
"sites": [
{ "name": "Site 1-1" },
{ "name": "Site 1-2" }
]
},
{
"name": "Category 2",
"sites": [
{ "name": "Site 2-1" },
{ "name": "Site 2-2" }
]
}
]
}
LeftNav.vue (左侧分类导航)
复制代码
<template>
<div class="left-nav">
<SearchBar @search="handleSearch" />
<ul v-if="categories">
<li v-for="(category, index) in categories" :key="index" @mouseenter="showSites(index)">
{{ category.name }}
<ExpandButton @click="expandCategory(index)" />
<div class="sites" v-if="activeIndex === index">
<ul>
<li v-for="(site, siteIndex) in category.sites" :key="siteIndex">{{ site.name }}</li>
</ul>
</div>
</li>
</ul>
</div>
</template>
<script setup lang="ts">
import { ref, onMounted } from 'vue';
import data from '../data.json';
import SearchBar from './SearchBar.vue';
import ExpandButton from './ExpandButton.vue';
const categories = ref(data.categories);
const activeIndex = ref(-1);
function handleSearch(searchTerm: string) {
// Implement search logic here
console.log('Searching for:', searchTerm);
}
function showSites(index: number) {
activeIndex.value = index;
}
function expandCategory(index: number) {
if (categories.value[index].expanded !== undefined) {
categories.value[index].expanded = true;
} else {
categories.value[index].expanded = false;
}
}
onMounted(() => {
// Initialization logic here
});
</script>
<style scoped>
.left-nav {
width: 200px;
padding: 10px;
background-color: #f4f4f4;
}
ul {
list-style: none;
padding: 0;
}
li {
position: relative;
padding: 5px 0;
cursor: pointer;
}
.sites {
display: none;
position: absolute;
top: 100%;
left: 0;
background-color: white;
border: 1px solid #ccc;
padding: 5px;
z-index: 10;
}
li:hover .sites {
display: block;
}
</style>
SearchBar.vue (搜索框)
复制代码
<template>
<input type="text" v-model="searchTerm" placeholder="Search..." @input="handleInput" />
</template>
<script setup lang="ts">
import { ref } from 'vue';
const searchTerm = ref('');
const emit = defineEmits(['search']);
function handleInput() {
emit('search', searchTerm.value);
}
</script>
<style scoped>
input {
width: 100%;
padding: 5px;
margin-bottom: 10px;
}
</style>
ExpandButton.vue (展开按钮)
复制代码
<template>
<button @click="handleClick">Expand</button>
</template>
<script setup lang="ts">
const emit = defineEmits(['click']);
function handleClick() {
emit('click');
}
</script>
<style scoped>
button {
margin-left: 5px;
}
</style>
App.vue (主应用)
复制代码
<template>
<div class="app">
<LeftNav />
<iframe src="about:blank" name="contentFrame"></iframe>
</div>
</template>
<script setup lang="ts">
import LeftNav from './components/LeftNav.vue';
</script>
<style scoped>
.app {
display: flex;
}
.left-nav {
flex: 1;
}
iframe {
flex: 2;
height: 100vh;
border: none;
}
</style>
main.ts (入口文件)
复制代码
import { createApp } from 'vue';
import App from './App.vue';
const app = createApp(App);
app.mount('#app');
这个示例代码是一个简单的实现,你可以根据需要进一步优化和扩展。如果你有任何问题或需要进一步的帮助,请点击【🔍追问按钮】提交补充信息。
我要提问
复制内容
分享给好友
AI编程问答网 免责声明:
以上内容除特别注明外均来源于网友提问,AI编程问答网回答,权益归原著者所有;